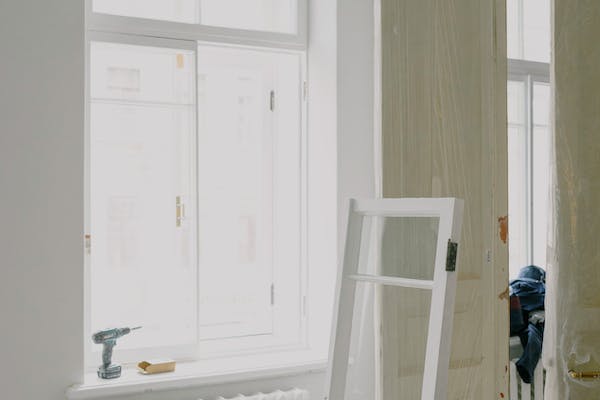
Unlocking the Power of Extension Objects: The Easy, Elegant, and Flexible Approach in Go
Introduction The extension object pattern is a clever way to add new features to an existing object without...
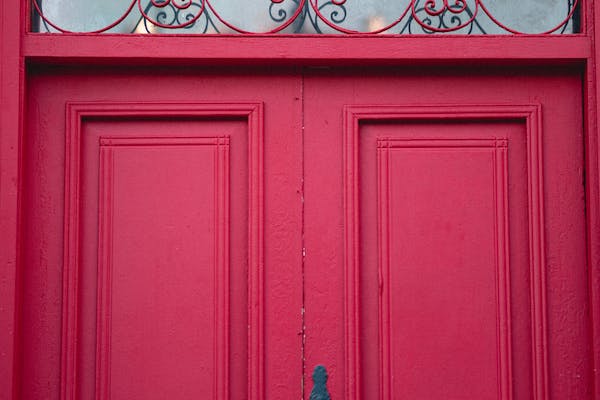
Easy delegation in Go: the delegation pattern
Introduction In delegation, you delegate a certain request to an object to a second object, which we call...
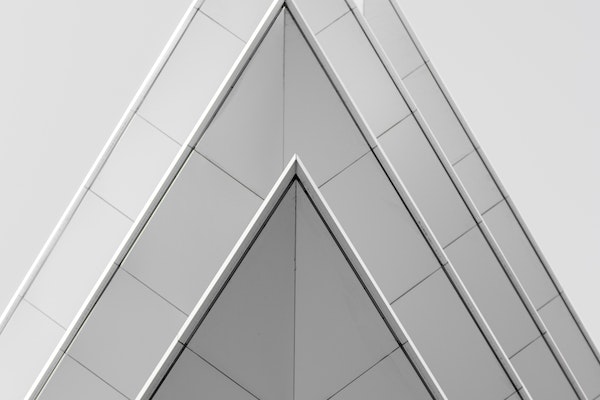
Elegant and Easy: Unleashing the Power of the Composite Pattern in Go
Introduction The composite pattern allows you treat a group of objects like a single object. The objects are...
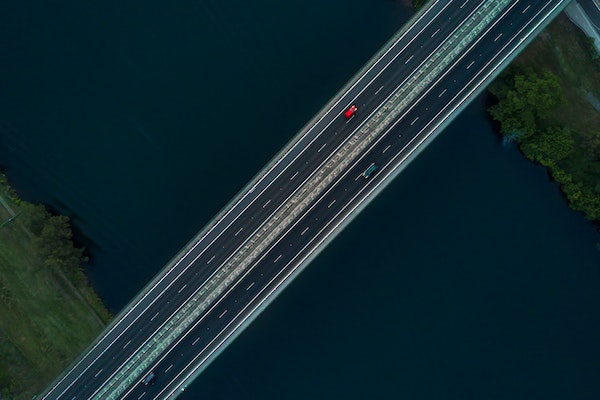
Easy Patterns in Go: The Bridge
Introduction The Bridge pattern is a design pattern that is meant to “decouple an abstraction from its implementation...
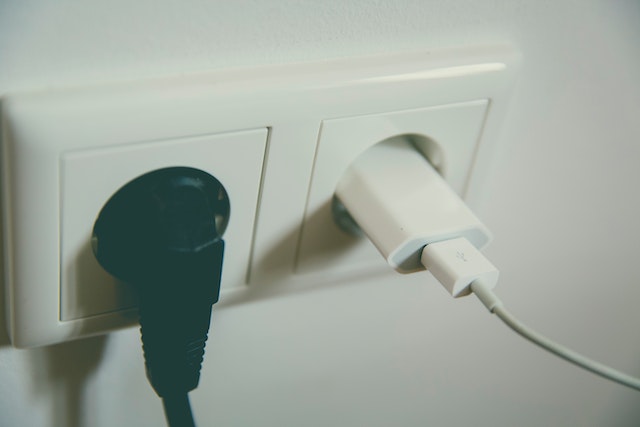
Easy patterns in Go: The Adapter Pattern
Introduction The Adapter pattern is used to make one interface compatible with another. It allows objects with different,...
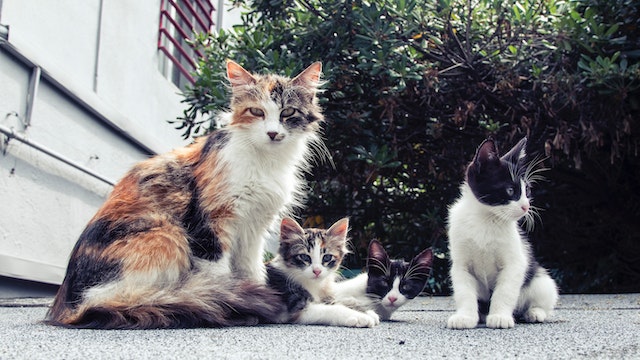
Using generics for implementing the Object Pool Pattern in Go
Introduction In my previous post I described simple implementation of the Object Pool pattern. In this post I...
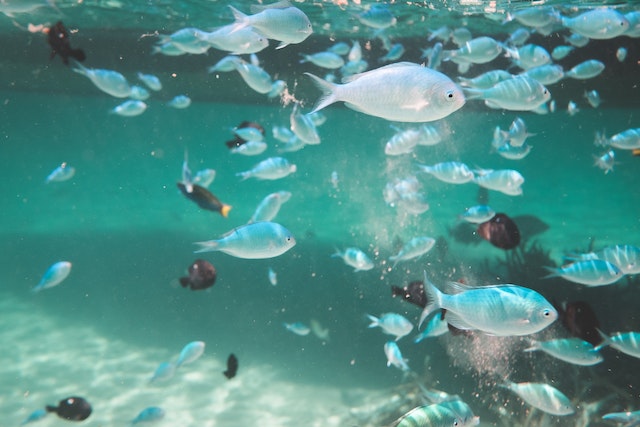
Optimizing Go Performance: implementing a threadsafe Object Pool
Introduction Sometimes, for reasons of efficiency, it can be quite handy to keep a pool, that is non-empty...
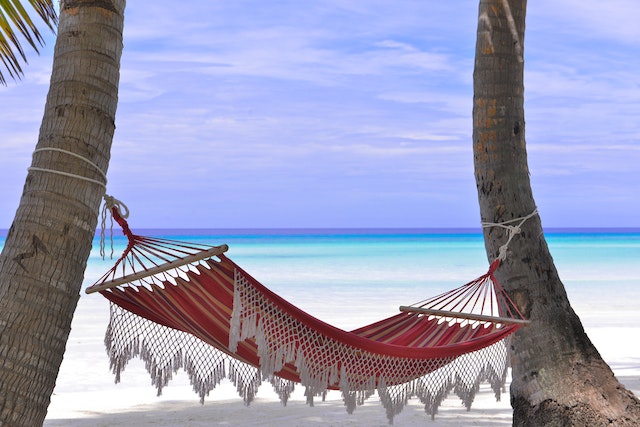
Lazy initialization and multiton: a cheap way of creating expensive objects
Sometimes creating an object is expensive, either an object takes up a lot of resources, or costs time...
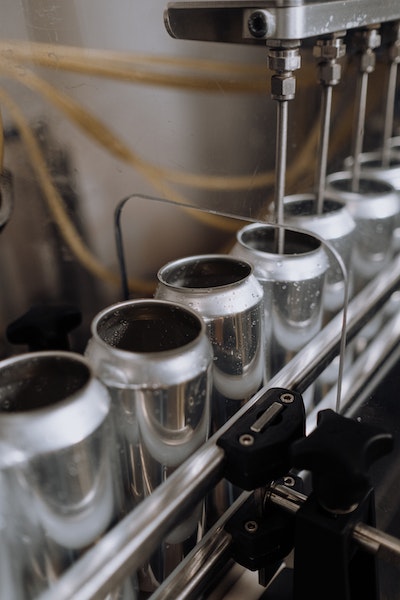
Design Patterns in Go: Factory method, automating the factory
Introduction In this article I discussed the implementation of the Abstract Factory pattern. The Factory Method is simply...
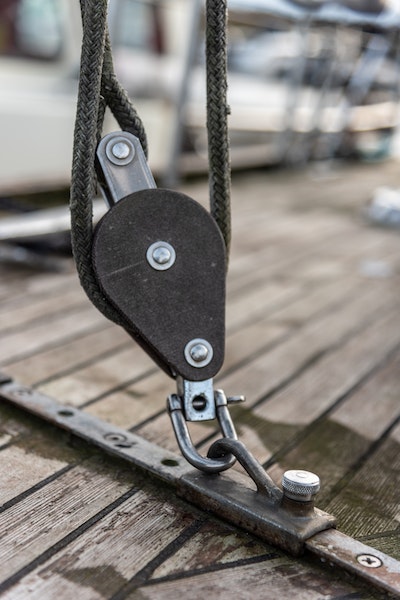
A simple way of implementing the Dependency Injection Pattern in Go
Introduction Dependency Injection is simply said, the idea that your classes should depend on abstraction, i.e. the abstraction...