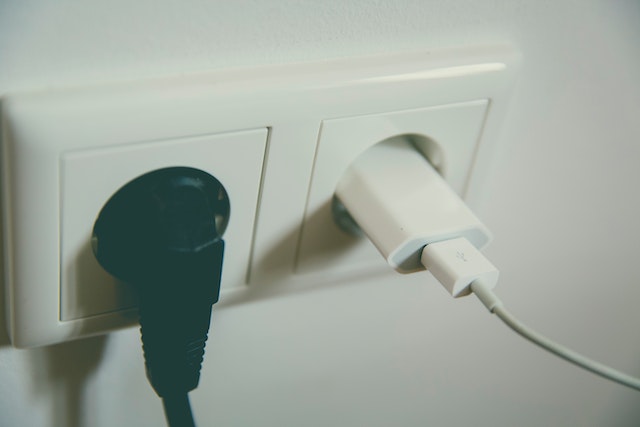
Easy patterns in Go: The Adapter Pattern
Introduction The Adapter pattern is used to make one interface compatible with another. It allows objects with different,...
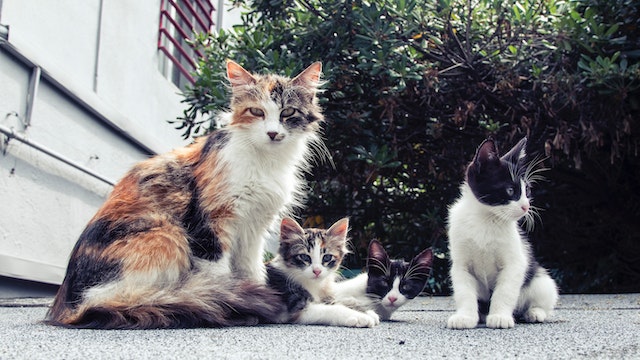
Using generics for implementing the Object Pool Pattern in Go
Introduction In my previous post I described simple implementation of the Object Pool pattern. In this post I...
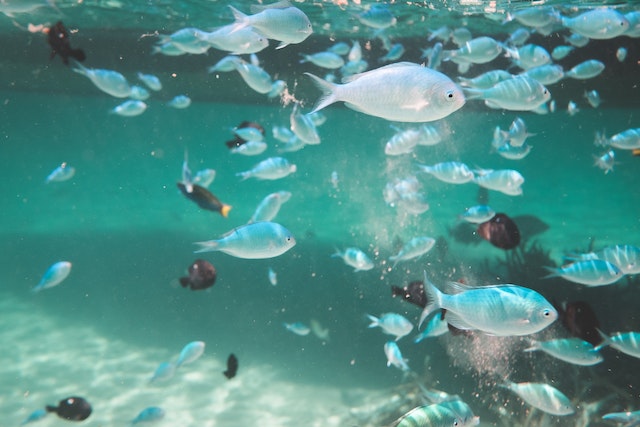
Optimizing Go Performance: implementing a threadsafe Object Pool
Introduction Sometimes, for reasons of efficiency, it can be quite handy to keep a pool, that is non-empty...
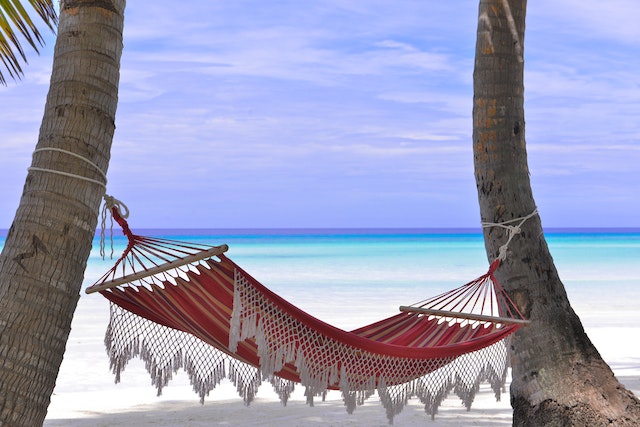
Lazy initialization and multiton: a cheap way of creating expensive objects
Sometimes creating an object is expensive, either an object takes up a lot of resources, or costs time...
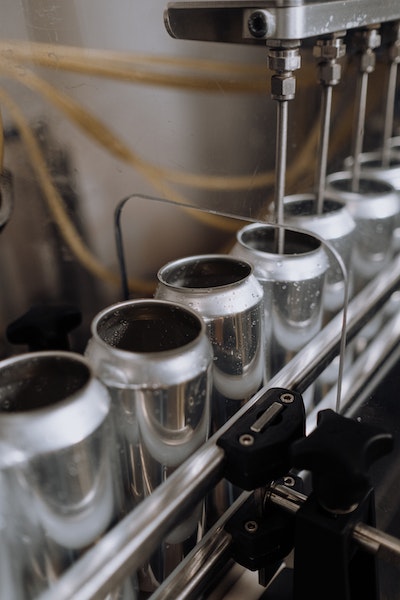
Design Patterns in Go: Factory method, automating the factory
Introduction In this article I discussed the implementation of the Abstract Factory pattern. The Factory Method is simply...
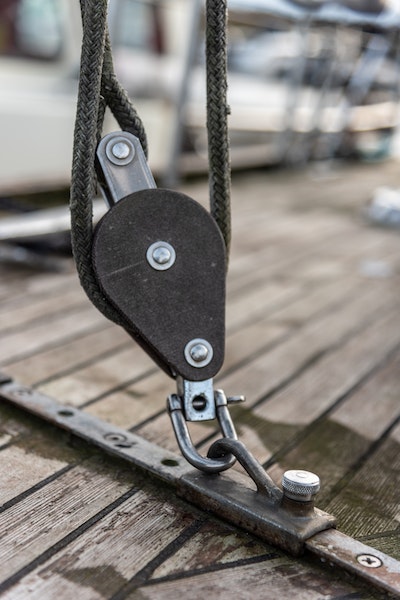
A simple way of implementing the Dependency Injection Pattern in Go
Introduction Dependency Injection is simply said, the idea that your classes should depend on abstraction, i.e. the abstraction...
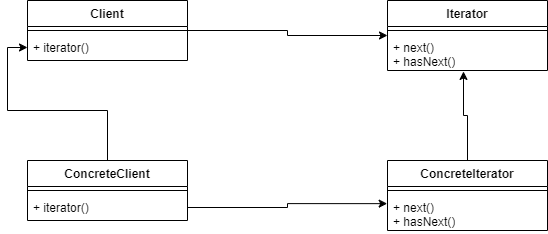
Design Patterns in Go: Using Iterator to traverse our containers
Introduction In Design Patterns, the Iterator is a way of traversing over a container, that is access each...
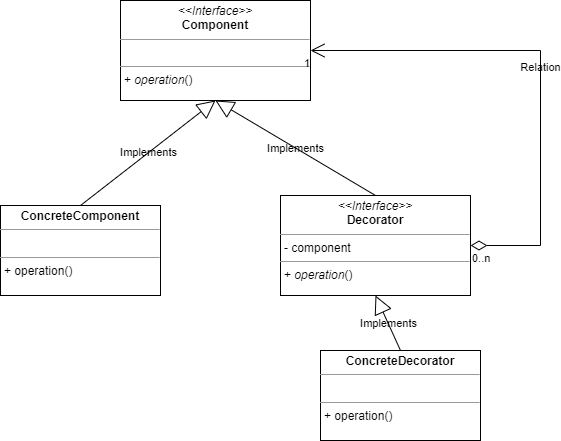
The Decorator pattern: an easy way to add functionality
Introduction The Decorator pattern can be used to dynamically alter or add functionality to existing classes. This pattern...
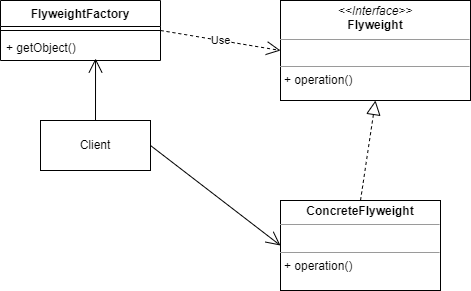
Design Patterns in Go: Flyweight, or go easy on your memory
Introduction The flyweight pattern is a pattern that helps minimize memory usage by sharing and reusing data. A...
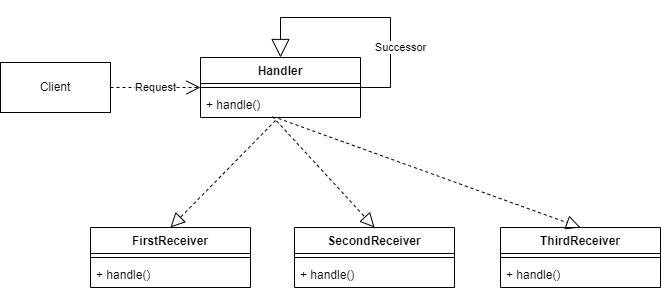
Design Patterns in Go: Chain of Responsibility: there is more than one way to do it
Introduction The Chain of Responsibility (CoC) pattern describes a chain of command/request receivers. The client has no idea...