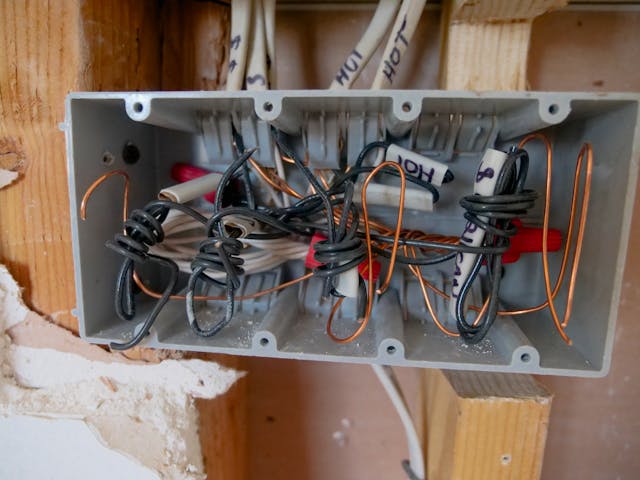
Easy Concurrency: Harnessing the Reactor Pattern in Go Programming
Introduction There are many ways to handle incoming events. If you need to be able to handle many...
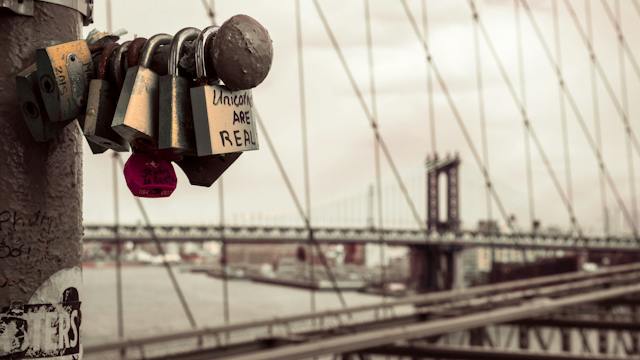
Simple Double Checked Locking in Go for Effortless Concurrency Control
Introduction Sometimes when locking data or objects it can be handy to reduce the overhead of acquiring a...
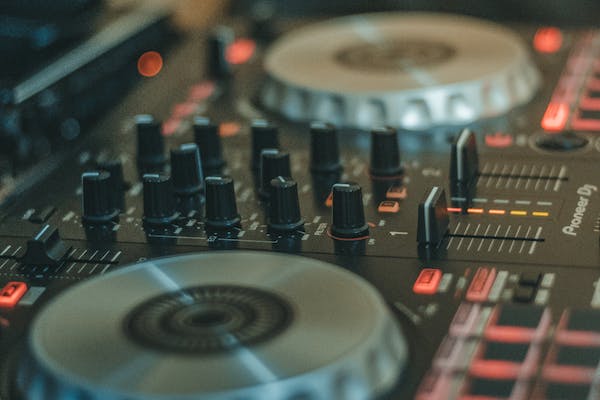
Demystifying Concurrency: Simple Implementation of the Binding Properties Pattern in Go
Introduction Especially in multi-threaded applications it can be necessary to synchronize properties between objects, or at least be...
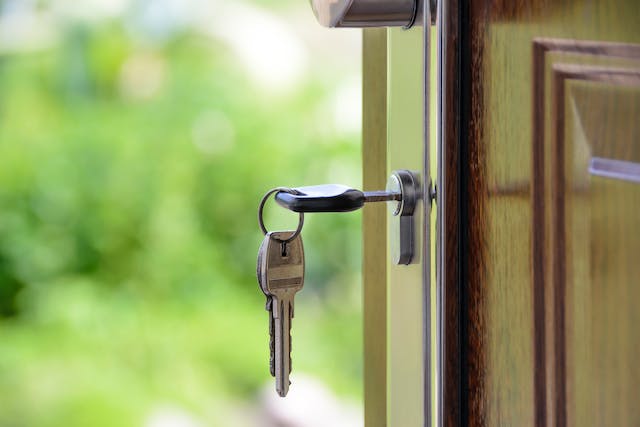
Unlocking Simplicity: Easy Concurrency with the Lock Design Pattern in Go
Introduction When we build programs that do many things at once, we want to make sure they’re secure....
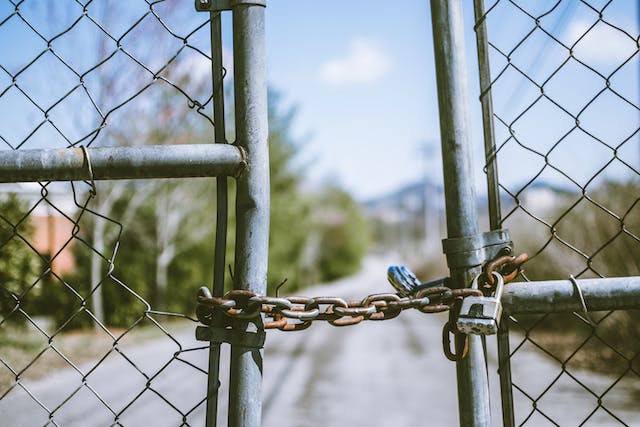
Mastering Concurrent Harmony: Easy Implementation of the Guarded Suspension Pattern in Go
Introduction In multithreaded applications, it’s common for one thread to let another know when specific conditions are met,...
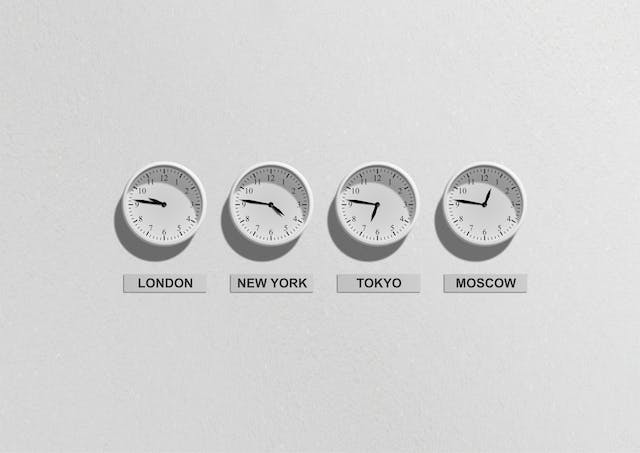
Mastering Go’s Event-Driven Brilliance: A Journey to Easy Asynchronous Excellence
Introduction Sometimes, when your program has a task that takes a lot of time, like working with databases,...
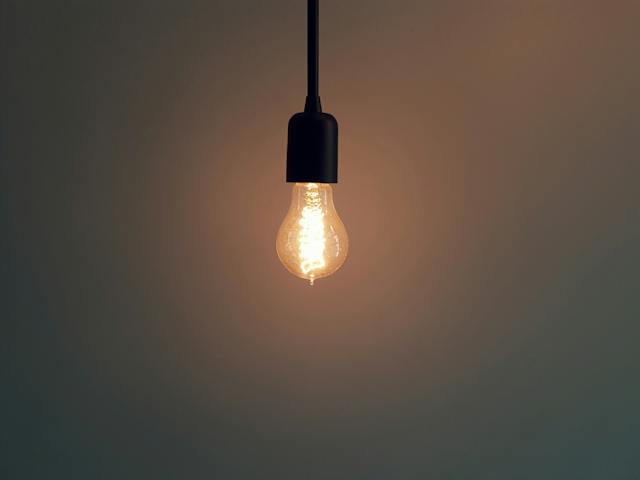
Easy Concurrency: Active Object Pattern in Go Explained
Introduction Sometimes you need to decouple method execution from method invocation. In such cases the Active Object design...
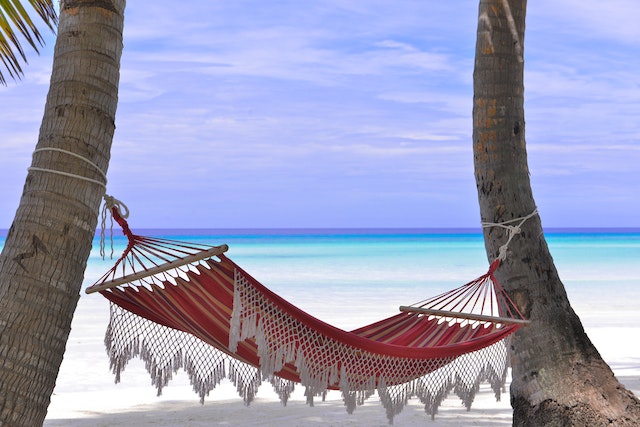
Lazy initialization and multiton: a cheap way of creating expensive objects
Sometimes creating an object is expensive, either an object takes up a lot of resources, or costs time...
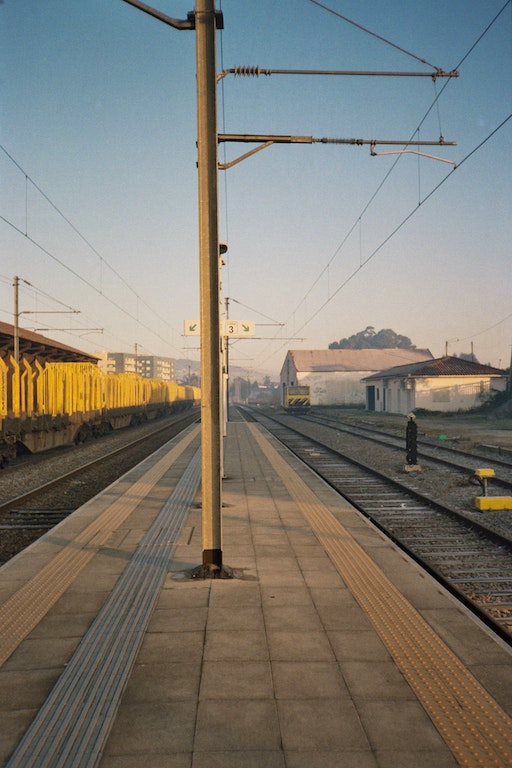
Building a web api with Go coroutines
Introduction On this page I described building a simple web API with a Postgres database at the backend....
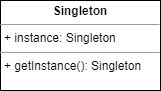
Design Patterns in Go: Singleton, a unique way of creating objects in a threadsafe way
Introduction The singleton pattern restricts the instantiation of a class to a single instance. The singleton pattern makes...