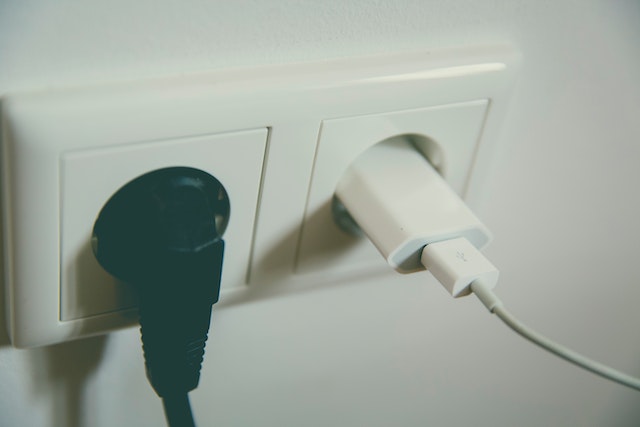
Easy patterns in Go: The Adapter Pattern
Introduction The Adapter pattern is used to make one interface compatible with another. It allows objects with different,...
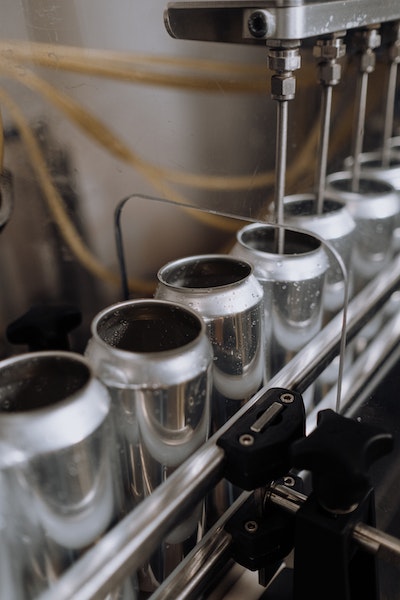
Design Patterns in Go: Factory method, automating the factory
Introduction In this article I discussed the implementation of the Abstract Factory pattern. The Factory Method is simply...
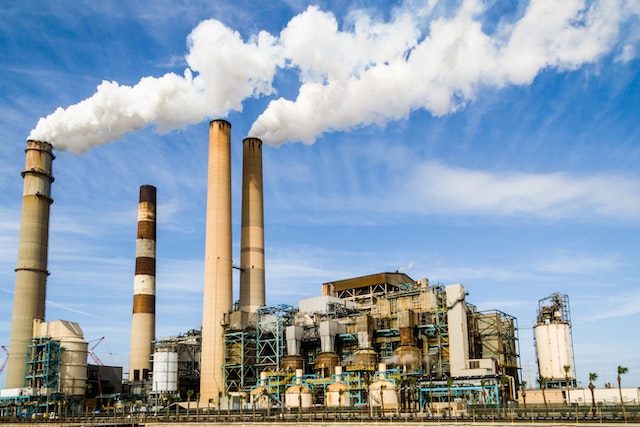
Design Patterns in Go: Abstract Factory, the flexible production of objects
Introduction The Abstract Factory Pattern is a way to group the creation of related objects, like products of...
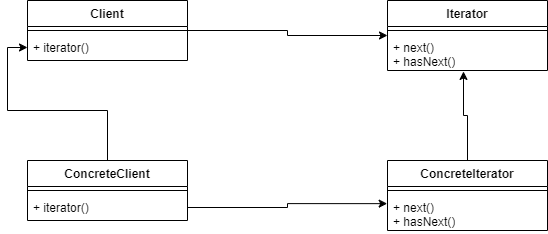
Design Patterns in Go: Using Iterator to traverse our containers
Introduction In Design Patterns, the Iterator is a way of traversing over a container, that is access each...
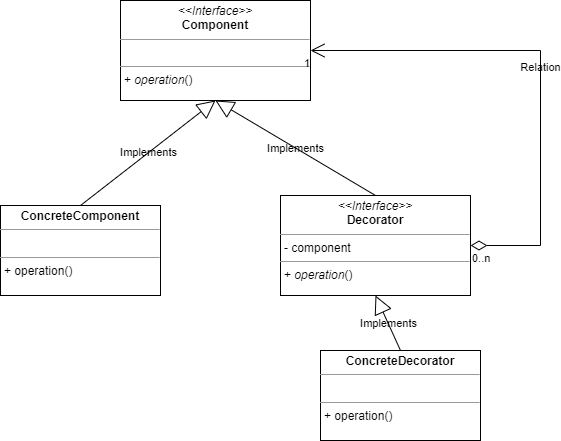
The Decorator pattern: an easy way to add functionality
Introduction The Decorator pattern can be used to dynamically alter or add functionality to existing classes. This pattern...
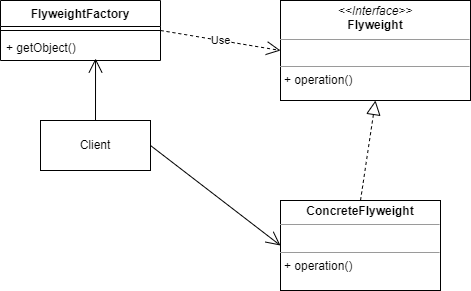
Design Patterns in Go: Flyweight, or go easy on your memory
Introduction The flyweight pattern is a pattern that helps minimize memory usage by sharing and reusing data. A...
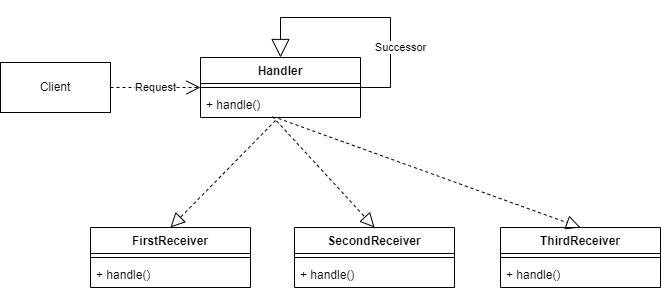
Design Patterns in Go: Chain of Responsibility: there is more than one way to do it
Introduction The Chain of Responsibility (CoC) pattern describes a chain of command/request receivers. The client has no idea...
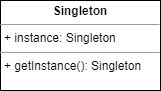
Design Patterns in Go: Singleton, a unique way of creating objects in a threadsafe way
Introduction The singleton pattern restricts the instantiation of a class to a single instance. The singleton pattern makes...
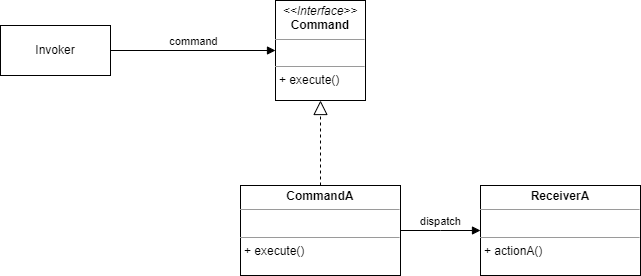
Design Patterns in Go: The Command, a simple implementation of a versatile pattern
Introduction The command pattern is a behavioral design pattern. It is used by an Invoker to perform one...
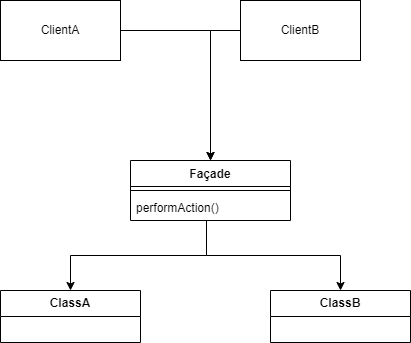
Design Patterns in Go: Facade, hiding a complex world
Introduction The facade pattern is used as a way to hide more complex logic. A facade can do...