In my previous article we set up an Azure Container App with a small web API in Go. In this article I will discuss adding CD to your App. That means that you can deploy directly from Github without having to go through building a docker image, pushing it and then pulling it again in your app.
Set up Managed Identity
In order to set up access rights correctly we need to set up Managed Identity. To do that, go to the Azure Container App we created in our previous article:
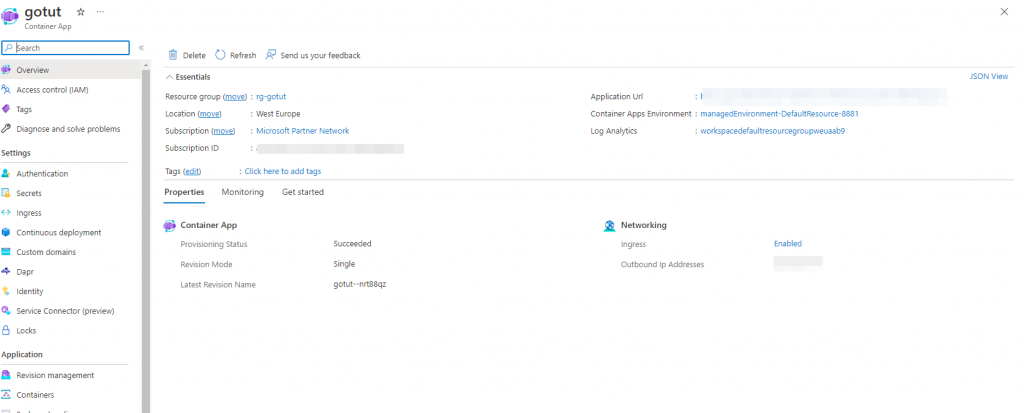
From this screen, click the ‘Identity’ item in the left pane:
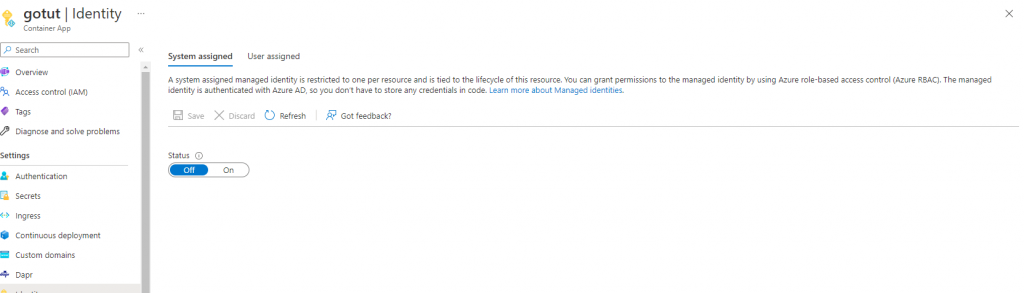
Set the status to ‘On’ and click ‘Save’:
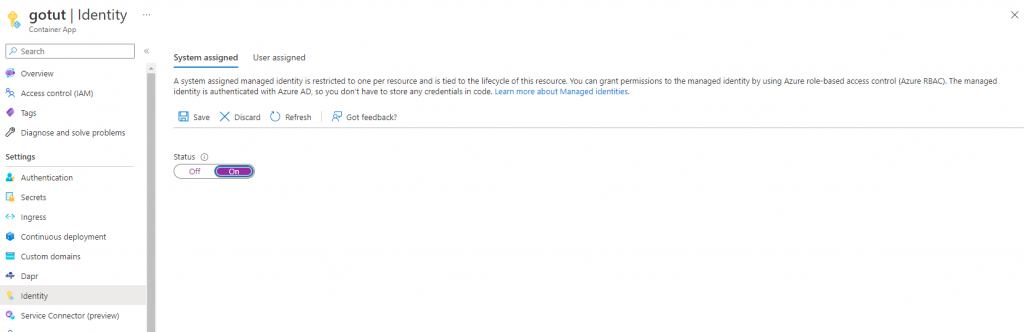
After a while you will be greeted by this screen:
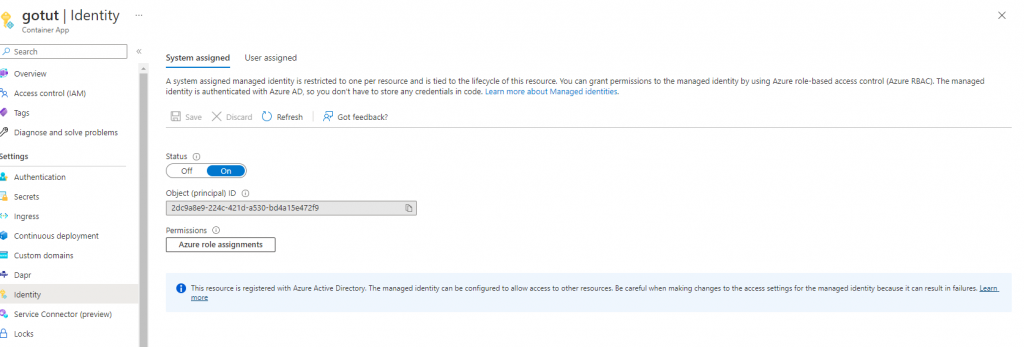
That’s it. Now we can go on to setting up our github repository.
Setting up Git
First log in to Github, you will see the following screen:
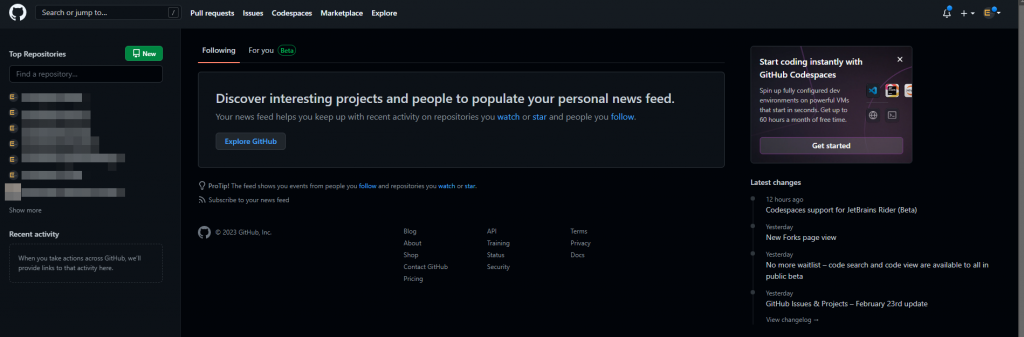
Click on the ‘New’ and you will see the following screen:
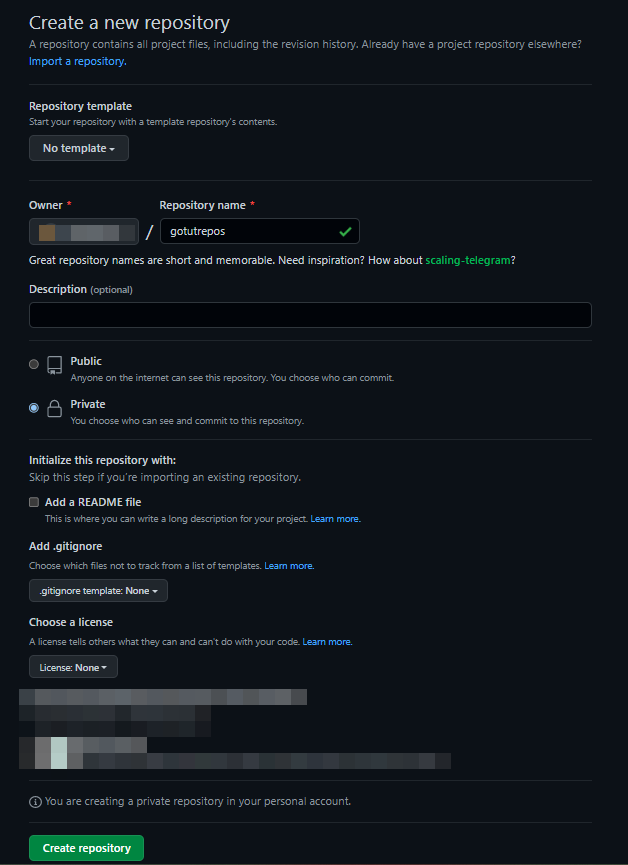
Enter ‘gotutrepos’ in the name field, and make it private. You can also make it public, but then anyone can see it, it depends on what you want. I chose private here.
After filling this in, click on ‘Create repository’ and you will be presented with the following screen:
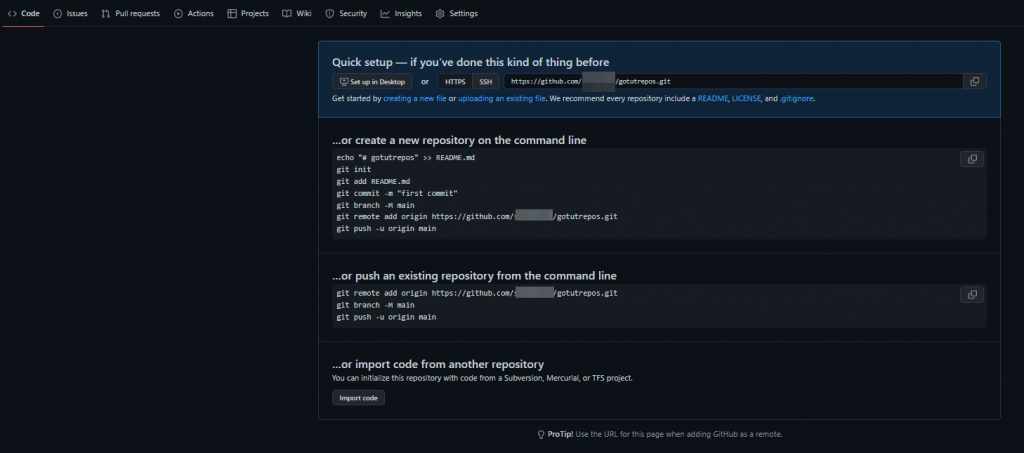
Now open a terminal or commandline in the directory where you orginally put the go tutorial files and enter
git init
git add *
git status
This will initialize a local git repository, add the files, and show you which files be committed. Now enter:
git commit -m 'first commit'
This will do a local commit. Now switch to the main branch
git branch -m main
And add the remote
git remote add origin https://github.com/<your githubusername>/gotutrepos.git
Now push the code to the server:
git push -u origin main
Now if you click on the Code tab, in the top left of the screen, you will see a screen like this
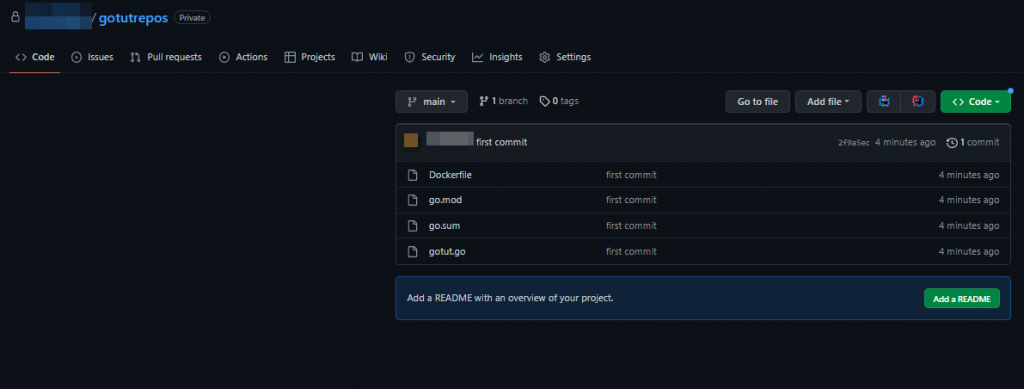
Congratulations! We have set up Git for our repository. The next step is to integrate this with our Azure Container App
Integration with the Azure Container App
Go back to Azure and into our Azure Container App
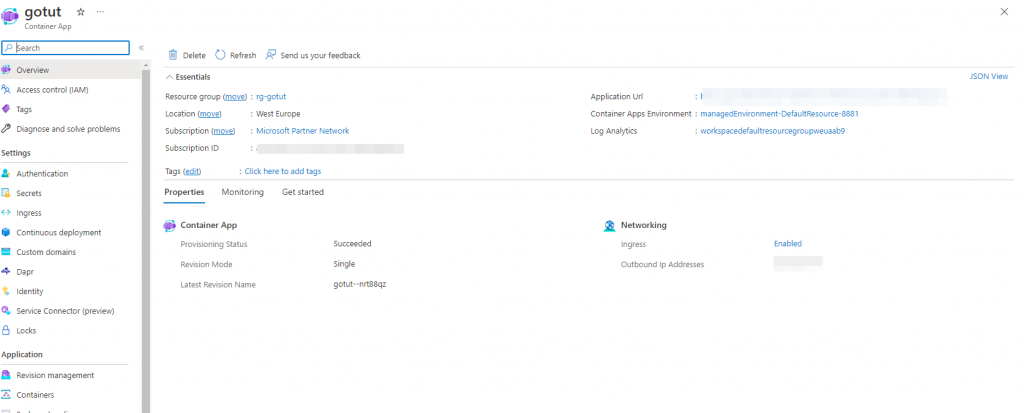
Now in the left pane, click on ‘Continuous Deployment’:
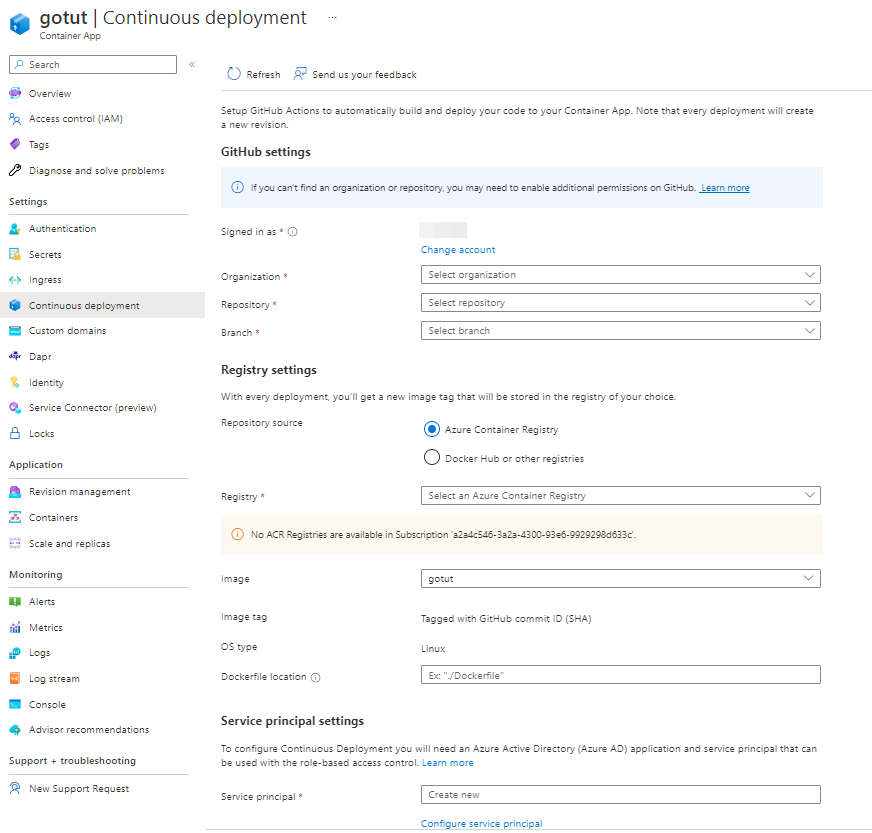
Enter the following:
- Make sure you are signed in with the right credentials. If not you can change them here.
- Choose the right organization, usually this equals your github username
- The repository is ‘gotutrepos’, and the branch is ‘main’
- Choose ‘Docker hub or other registries’
- The image name is ‘gotut’
- Login server URL is docker.io
- Enter your docker hub username.
- Enter your docker hub password. The password will become a github pipeline secret and will never be visible in any code
- Leave the Dockerfile location empty, it will find it automatically
Now click on ‘Start continuous deployment’
Once that is finished, go back to github and click the ‘Actions’ tab. You will see a pipeline action has been started:
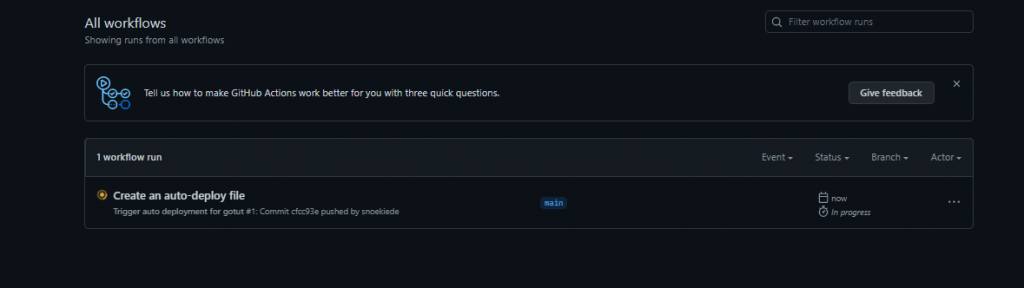
Once that has finished, go to your dockerhub, and look in your gotut repository:
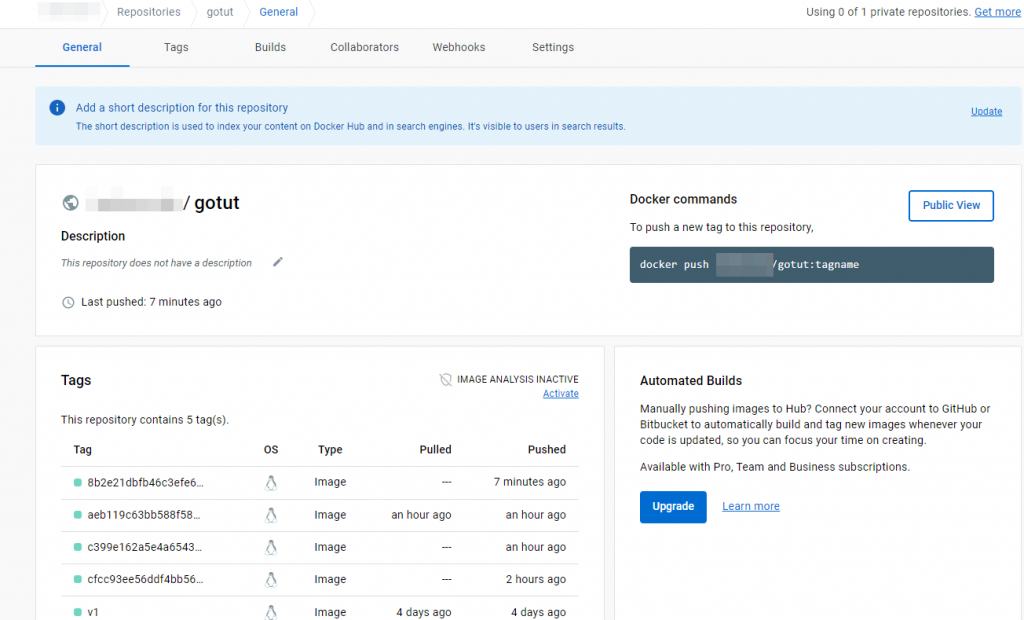
You will see a new tag appear, with the sha of the git build (this looks like quite a random number to me).
Now go back to your gotut container app in the Azure portal, and click the ‘Revision Management’ in the left pane. You should see something similar to this:
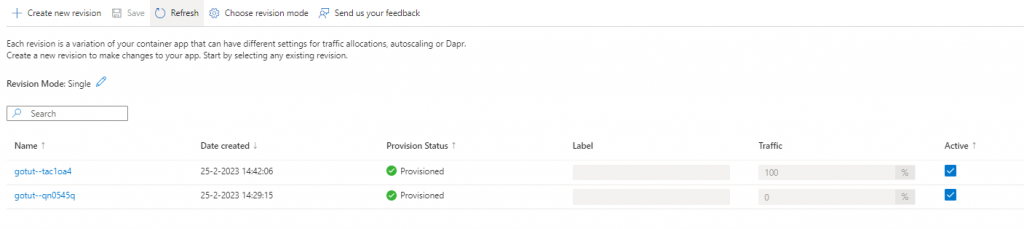
It could well be that you only see one revision, the Revision mode has been set to single, so old revisions are removed. What matters is the time stamp, that should be close to your current time.
Putting it to the test
Now it is time to put the CD-pipeline to the test. For us to do that, open your Visual Studio Code in your gotut directory, you should see something like this:
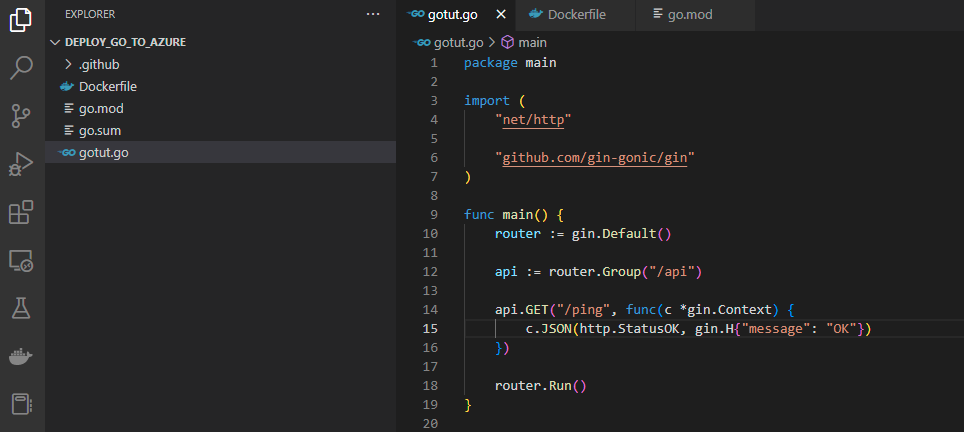
Now change line 15:
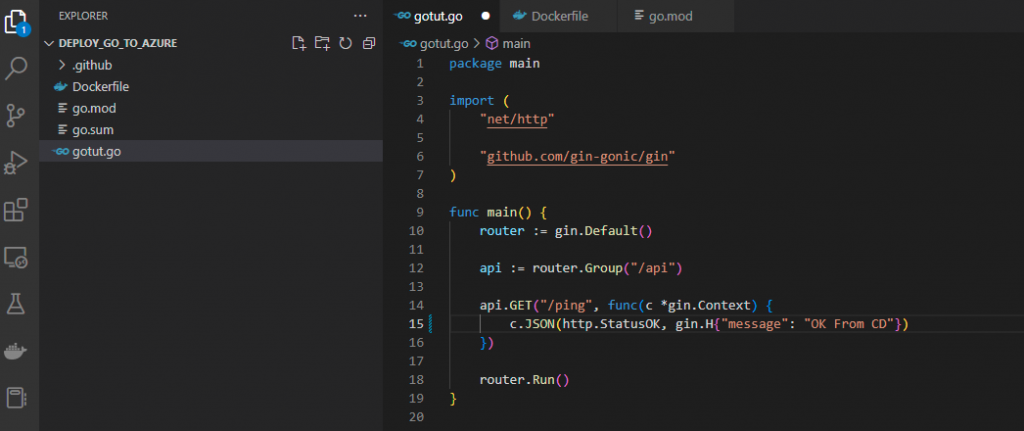
Save this file either by using File->Save All in the menu or Ctrl S/Cmd S. Then your VS Code should look like this:
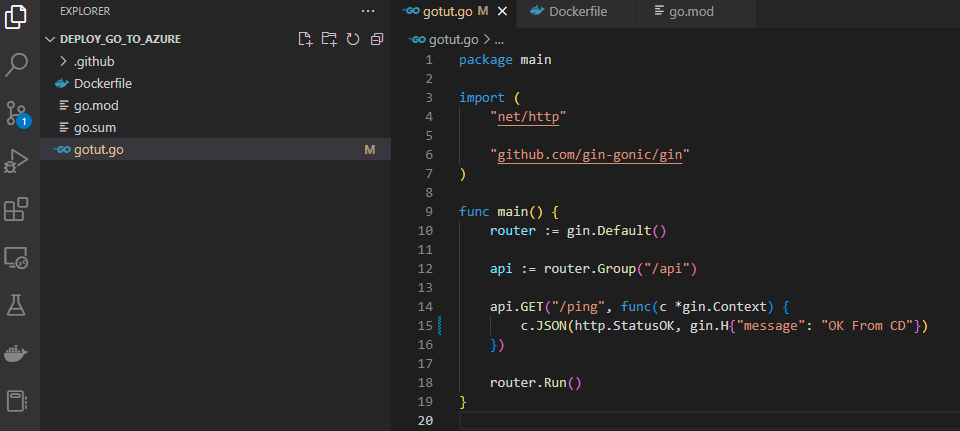
Now click on the Source Control Icon (where the little 1 appeared), and VS Code should look like this:
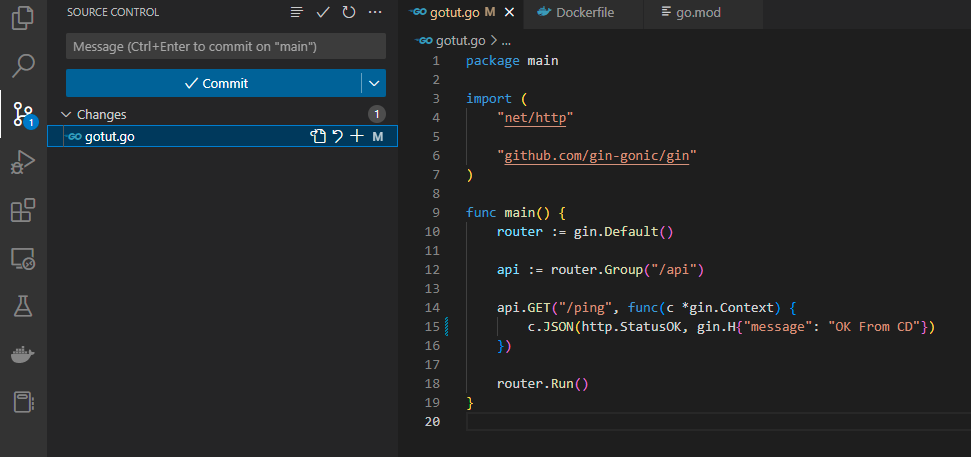
Now type a message in the Messagebox in the left top, and click ‘Commit’:
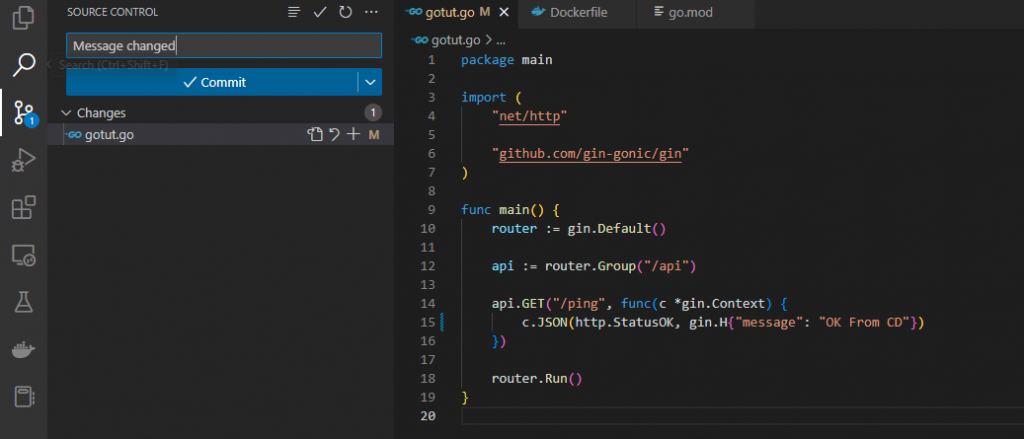
You are now making a local commit, nothing will happen now. Once you push the code to the server, the pipeline will start. To do that click on ‘Sync Changes’:
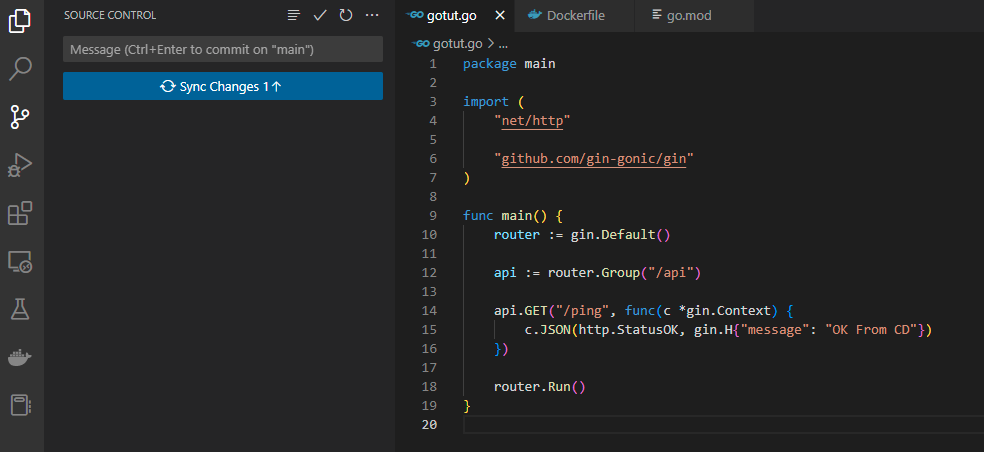
Go back to your project on Github, and click the actions tab, you will see something like this:

And if you click the action, you should see something like this:
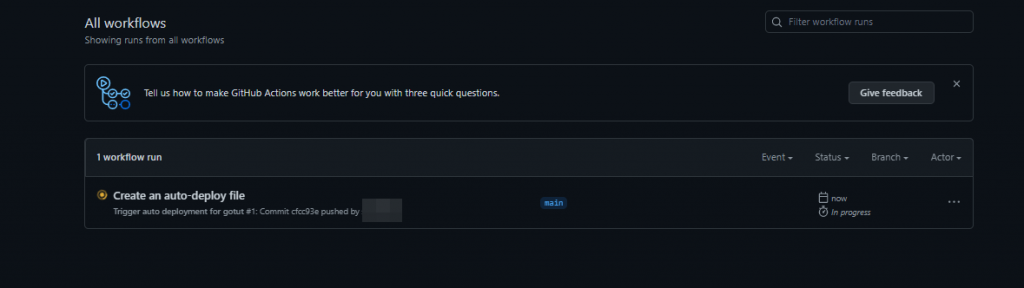
If you want to know more about the progress of the pipeline you can click the pipeline, and you will get information.
Wait till the pipeline has finished, then go to your gotut Container App in the Azure Portal, and try it out

Use the application URL in the top right corner and copy it using the icon to the right of it. Now paste it in a browser and add /api/ping to it, and if everything went well, you should see this:
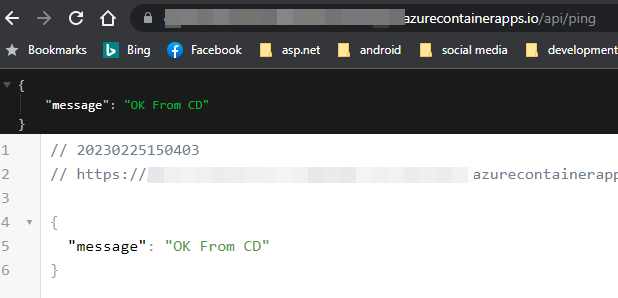
That is it, you have set up a simple pipeline for continously deploying your go web api.